Page script / toolbar button example
The page script / toolbar button example adds a button to the toolbar on the Offenses or Offense Summary tabs in IBM® QRadar®. The new button calls a page script when you click it.
When you select an offense in the Offenses or Offense Summary tab, and click the button, information about the selected offense is displayed in an alert.
The following image shows the My Offense Button.
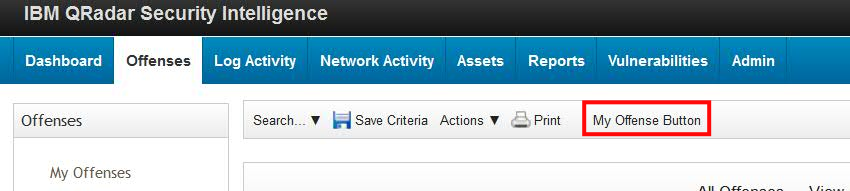
The following image shows the output from using the My Offense Button.

The following table shows the files that are used for this sample app:
Files/Folders | Description |
---|---|
app | The root directory for application files. The app folder contains the following files:
qpylib folder that contains the Python library files that your application can use to connect with QRadar API endpoints. __init__.py - a sample initialization file your app. It creates a Flask instance, imports views from views.py and functions from the qpylib library. views.py - The main entry point into the web application. This file and the manifest.json file are the only files that are required in every application. This file contains sample code for the sample application. The /static/js/custom_script.js script that is run when the button is clicked. |
__init__.py | Creates an instance of the Flask micro-framework that is used to serve content to QRadar. |
manifest.json | This file tells QRadar what the sample app does. |
manifest.json
The manifest.json file contains the following code:
{
"name":"Page script test App",
"description":"An example of to test page scripts",
"version":"1.0.1",
"uuid":"4a5d50cc-b9f1-4526-b356-5cb2d60e9467",
"rest_methods": [
{
"name":"offenseListFunction",
"url":"/offenseListFunction",
"method":"GET",
"argument_names":["appContext"]
}
],
"gui_actions": [
{
"id":"OffenseListToolbarButton",
"text":"My Offense Button",
"description":"My Offense Button",
"icon":"",
"rest_method":"offenseListFunction",
"javascript":"my_offense_toolbar_button_action(result)",
"groups":["OffenseListToolbar"]
},
{
"id":"OffenseSummaryToolbarButton",
"text":"My Offense Button",
"description":"My Offense Button",
"icon":"",
"rest_method":"offenseListFunction",
"javascript":"my_offense_toolbar_button_action(result)",
"groups":["OffenseSummaryToolbar"]
}
],
"page_scripts": [
{
"app_name":"SEM",
"page_id":"OffenseList",
"scripts":["static/js/custom_script.js"]
},
{
"app_name":"SEM",
"page_id":"OffenseSummary",
"scripts":["static/js/custom_script.js"]
}
],
}
The first three objects, name
, description
, and
version
, provide basic app information.
The gui_actions
object describes a new GUI Action that the user can perform
in the QRadar user interface.
It is abstracted from the underlying representation.
GUI Actions are represented as buttons on page toolbars, or as right-click menu options.
GUI Actions can run a block of JavaScript, invoke a REST method, or both.
The gui_actions
block contains sections for both the Offenses
page toolbar and the Offense Summary page toolbar.
The Offenses page toolbar section contains the fields that are described in the following table:
Field | Description | Value |
---|---|---|
id | A unique ID for this area within the application | OffenseListToolbarButton |
text | The name of the GUI Action that is displayed in the user interface. | My Offense Button |
description | A description of the GUI Action that is displayed when your mouse hovers over the item. | My Offense Button |
icon | A URL to load, relative to the application root. Only URLs that exist within the app can be referenced. | "" |
rest_method | The name of the REST method to load this item. This method must be declared in the rest_methods block of the manifest. | offenseListFunction |
javascript | A JavaScript code block to run when this action is performed. Either this argument or the
REST method argument is required. If both are specified, then the REST method is run first, the
results are then passed into the JavaScript code block by using the variable
result. If only the JavaScript argument is specified, a context variable that contains the context of the GUI Action, is passed into the JavaScript code block. |
|
groups | A list of one or more GUI Action groups to install the action into. This string is the
identifier of the toolbar or right-click menu in QRadar. At least one group must be
provided. You can also use a group name in this format
|
["OffenseListToolbar"] |
The Offenses Summary page toolbar section contains the fields that are described in the following table:
Field | Description | Value |
---|---|---|
id | A unique ID for this area within the application | OffenseSummaryToolbarButton |
text | The name of the GUI Action that is displayed in the user interface. | My Offense Button |
description | A description of the GUI Action that is displayed when your mouse hovers over the item. | My Offense Button |
icon | A URL to load, relative to the application root. Only URLs that exist within the QRadar app can be referenced. | "" |
javascript | A JavaScript code block to run when this action is performed. Either this argument or the
REST method argument is required. If both are specified, then the REST method is run first, the
results are then passed into the JavaScript code block by using the variable
result. If only the JavaScript argument is specified, a context variable that contains the context of the GUI Action, is passed into the JavaScript code block. |
|
groups | A list of one or more GUI Action groups to install the action into. This string is the
identifier of the toolbar or right-click menu in QRadar. At least 1 group must be
provided. You can also use a group name in this format
|
["OffenseSummaryToolbar"] |
The rest_methods
block contains the fields that are described in the
following table:
Field | Description | Value |
---|---|---|
name | A unique name for this REST method within the application. | offenseListFunction |
url | The URL to access the REST method, relative to the application root. Only URLs within the app are supported. | /offenseListFunction |
method | The HTTP method on the named endpoint (GET/POST/DELETE). | GET |
argument_names | The names of arguments that this method expects. Arguments that are passed to the method URL are encoded, as either query string parameters or in the PUT/POST body. | ["context"] |
The page_scripts
block contains the fields that are described in the
following table:
Name | Description | Value |
---|---|---|
app_name | The name of the QRadar application you want to include the script into. The wildcard "*" is also supported if it is used with the page_id field, to have a file included in every QRadar page. | SEM |
page_id | The ID of the QRadar page where the script is included. The wildcard "*" is also supported when it is used with the app_namefield. When the wildcard character is used, a file is included on every QRadar page. | OffenseList |
scripts | The scripts that you want to include, relative to the application root. | ["static/js/custom_script.js"] |
views.py
The views.py for this example establishes the Flask routes that are used by the application. The script injects context information from the REST API endpoint into the offense variable. This content is used by the page script.
__author__ = 'IBM'
from flask import request
from app import app
from qpylib import qpylib
import json
@app.route('/offenseListFunction', methods=['GET'])
def offenseListFunction():
qpylib.log("offenseListFunction", "info")
offense = request.args.get("appContext")
qpylib.log("appContext=" + offense, "info")
#You can process the data and return any value here,
#that will be passed into javascript
return json.dumps({'context_passed_to_python_route':offense})
static/js/custom_script.js
Contains the function that turns the content of the offense variable into a string and displays it within a JavaScript alert dialog.
function my_offense_toolbar_button_action(offense)
{
alert(JSON.stringify(offense));
}
Script files must be fragments and not fully formed HTML because they are injected in to a fully rendered page.